Tips and tricks working with Shapes
In Xamarin.Forms 4.7 we have in the Xamarin.Forms.Shapes namespace support to draw shapes on the screen in Android, iOS, macOS, UWP and WPF.
We have from simple shapes to more complex options that allow us to practically draw whatever we want. But … how do we draw a specific shape?. In this article we are going to learn tips and tricks to draw shapes in Xamarin.Forms.
We have from simple shapes to more complex options that allow us to practically draw whatever we want. But … how do we draw a specific shape?. In this article we are going to learn tips and tricks to draw shapes in Xamarin.Forms.
From Design to Shapes
When developing mobile applications, some times sometimes we replicate a design. There is a huge variety of design tools such as Figma, Adobe XD or Sketch for example. In some cases there are plugins to export to XAML although what is available in all of them is the possibility of export to SVG format. Do you know that we can convert any SVG to a Xamarin.Forms Shape in a simple way?.
For example, let’s take a relatively complex SVG created with a composition of paths:
<svg viewBox='0 0 104 97' xmlns='http://www.w3.org/2000/svg'> <path d='M14,85l3,9h72c0,0,5-9,4-10c-2-2-79,0-79,1' fill='#7C4E32'/> <path d='M19,47c0,0-9,7-13,14c-5,6,3,7,3,7l1,14c0,0,10,8,23,8c14,0,26,1,28,0c2-1,9-2,9-4c1-1,27,1,27-9c0-10,7-20-11-29c-17-9-67-1-67-1' fill='#E30000'/> <path d='M17,32c-3,48,80,43,71-3 l-35-15' fill='#FFE1C4'/> <path d="M17,32c9-36,61-32,71-3c-20-9-40-9-71,3" fill="#8ED8F8"/> <path d='M54,35a10 8 60 1 1 0,0.1zM37,38a10 8 -60 1 1 0,0.1z' fill='#FFF'/> <path d='M41,6c1-1,4-3,8-3c3-0,9-1,14,3l-1,2h-2h-2c0,0-3,1-5,0c-2-1-1-1-1-1l-3,1l-2-1h-1c0,0-1,2-3,2c0,0-2-1-2-3M17,34l0-2c0,0,35-20,71-3v2c0,0-35-17-71,3M5,62c3-2,5-2,8,0c3,2,13,6,8,11c-2,2-6,0-8,0c-1,1-4,2-6,1c-4-3-6-8-2-12M99,59c0,0-9-2-11,4l-3,5c0,1-2,3,3,3c5,0,5,2,7,2c3,0,7-1,7-4c0-4-1-11-3-10' fill='#FFF200'/> <path d='M56,78v1M55,69v1M55,87v1' stroke='#000' stroke-linecap='round'/> <path d='M60,36a1 1 0 1 1 0-0.1M49,36a1 1 0 1 1 0-0.1M57,55a2 3 0 1 1 0-0.1M12,94c0,0,20-4,42,0c0,0,27-4,39,0z'/> <path d='M50,59c0,0,4,3,10,0M56,66l2,12l-2,12M25,50c0,0,10,12,23,12c13,0,24,0,35-15' fill='none' stroke='#000' stroke-width='0.5'/> </svg>
Draw the Cartman character from South Park. We can transform the previous code to XAML in a very simple way:
<Grid> <Path Data="M14,85l3,9h72c0,0,5-9,4-10c-2-2-79,0-79,1" Fill="#7C4E32"/> <Path Data="M19,47c0,0-9,7-13,14c-5,6,3,7,3,7l1,14c0,0,10,8,23,8c14,0,26,1,28,0c2-1,9-2,9-4c1-1,27,1,27-9c0-10,7-20-11-29c-17-9-67-1-67-1" Fill="#E30000"/> <Path Data="M17,32c-3,48,80,43,71-3 l-35-15" Fill="#FFE1C4"/> <Path Data="M17,32c9-36,61-32,71-3c-20-9-40-9-71,3" Fill="#8ED8F8"/> <Path Data="M54,35a10 8 60 1 1 0,0.1zM37,38a10 8 -60 1 1 0,0.1z" Fill="#FFF"/> <Path Data="M41,6c1-1,4-3,8-3c3-0,9-1,14,3l-1,2h-2h-2c0,0-3,1-5,0c-2-1-1-1-1-1l-3,1l-2-1h-1c0,0-1,2-3,2c0,0-2-1-2-3M17,34l0-2c0,0,35-20,71-3v2c0,0-35-17-71,3M5,62c3-2,5-2,8,0c3,2,13,6,8,11c-2,2-6,0-8,0c-1,1-4,2-6,1c-4-3-6-8-2-12M99,59c0,0-9-2-11,4l-3,5c0,1-2,3,3,3c5,0,5,2,7,2c3,0,7-1,7-4c0-4-1-11-3-10" Fill="#FFF200"/> <Path Data="M56,78v1M55,69v1M55,87v1" Stroke="#000" StrokeLineCap="Round"/> <Path Data="M60,36a1 1 0 1 1 0-0.1M49,36a1 1 0 1 1 0-0.1M57,55a2 3 0 1 1 0-0.1M12,94c0,0,20-4,42,0c0,0,27-4,39,0z" Stroke="#000"/> <Path Data="M50,59c0,0,4,3,10,0M56,66l2,12l-2,12M25,50c0,0,10,12,23,12c13,0,24,0,35-15" Stroke="#000" StrokeThickness="0.5"/> </Grid>
The result:
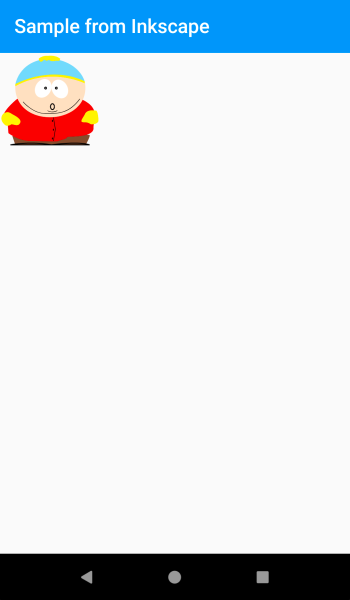
Dibujando usando SVG
Each Path draws an element of the character so we can apply transformations, animations, etc. to each small part that makes up the drawing. Ah, remember that XAML Hot Reload works with Shapes:

Usando Hot Reload
Drawing with tools
A nice tool for creating Shapes is Blend. Among the set of Assets you can directly drag and drop any type of Shape, and then do any transformation like rotate, scale, etc.

Shapes
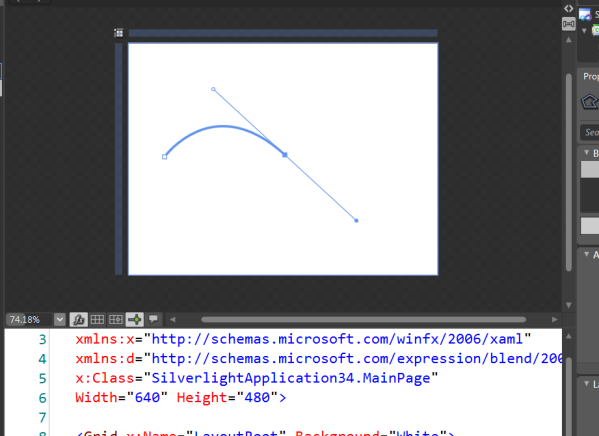
Dibujando Shapes con Blend
Another classic tool but that allows us to directly draw and export XAML code that we can use in our Xamarin.Forms application is Inkscape. Drawing tool available for Windows, macOS and Linux allows us a great variety of options (that we can extend using plugins) to draw:

Inkscape
And export the code directly to XAML:
<?xml version="1.0" encoding="UTF-8"?> <!--This file is NOT compatible with Silverlight--> <Viewbox xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation" Stretch="Uniform"> <Canvas Name="svg8" Width="210" Height="297"> <Canvas.RenderTransform> <TranslateTransform X="0" Y="0"/> </Canvas.RenderTransform> <Canvas.Resources/> <!--Unknown tag: sodipodi:namedview--> <!--Unknown tag: metadata--> <Canvas Name="layer1"> <Path xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" Name="path10" StrokeThickness="0.264583" Stroke="#FF000000"> <Path.Data> <PathGeometry Figures="m 130.77975 114.90475 c -1.28867 2.76686 -4.36546 -0.37204 -4.5987 -2.14187 -0.63208 -4.79613 4.79124 -7.58089 8.88244 -7.05555 7.31819 0.93971 11.05326 8.94515 9.51239 15.62302 -2.26128 9.80005 -13.14527 14.60051 -22.36359 11.96923 -12.28658 -3.50708 -18.17801 -17.36047 -14.42608 -29.10417 4.72141 -14.778243 21.58254 -21.770781 35.84475 -16.882919 17.27344 5.919857 25.37235 25.808419 19.33976 42.585329 -7.1092 19.771 -30.03665 28.97944 -49.3259 21.7966 C 91.374607 143.40155 81.054615 117.42797 89.391371 95.627942 98.864123 70.857366 127.88874 59.424405 152.19843 68.917654 c 27.27179 10.649991 39.81874 42.729096 29.16713 69.547636 -11.82531 29.77366 -46.96143 43.43535 -76.28821 31.62397" FillRule="EvenOdd"/ </Path.Data> </Path> </Canvas> </Canvas> </Viewbox>
So, we can use the result in our applications:
<Path StrokeThickness="0.264583" Stroke="#FF000000"> <Path.Data> <PathGeometry Figures="m 130.77975 114.90475 c -1.28867 2.76686 -4.36546 -0.37204 -4.5987 -2.14187 -0.63208 -4.79613 4.79124 -7.58089 8.88244 -7.05555 7.31819 0.93971 11.05326 8.94515 9.51239 15.62302 -2.26128 9.80005 -13.14527 14.60051 -22.36359 11.96923 -12.28658 -3.50708 -18.17801 -17.36047 -14.42608 -29.10417 4.72141 -14.778243 21.58254 -21.770781 35.84475 -16.882919 17.27344 5.919857 25.37235 25.808419 19.33976 42.585329 -7.1092 19.771 -30.03665 28.97944 -49.3259 21.7966 C 91.374607 143.40155 81.054615 117.42797 89.391371 95.627942 98.864123 70.857366 127.88874 59.424405 152.19843 68.917654 c 27.27179 10.649991 39.81874 42.729096 29.16713 69.547636 -11.82531 29.77366 -46.96143 43.43535 -76.28821 31.62397" FillRule="EvenOdd"/> </Path.Data> </Path>

El resultado
Other tools
There are a huge variety of tools and possibilities for working with Shapes. However, I would also like to recommend Metro Studio. It is a free SyncFusion tool that has more than 7000 different icons and shapes to export to XAML code. We can take advantage of this XAML code to create icons using Shapes and draw different shapes.
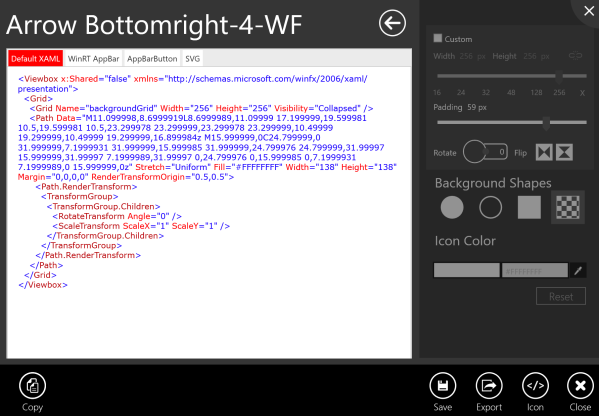
Metro Studio
Snippets
To facilitate the Shapes creation I created some snippets for Visual Studio. If you need guidance on how to implement snippets in Visual Studio 2019, head on over to the documentation guide.
Great !