It’s an unbelievably difficult time – we do hope everyone is doing well and making the best out of present circumstances. If we can be of help, please don’t hesitate to reach out (clientservices@devexpress.com). A big shout-out to all the brilliant scientists throughout the world focused on a vaccine/cure...Thank you for your hard work.
Each time our team implements a new feature, we do our best to describe its capabilities. In our zeal to document popular controls such as our WinForms Data Grid, we often fail to articulate the power and flexibility of the utility controls included in our WinForms Subscription.
Message Boxes are a perfect example of high-value utility controls within our WinForms product portfolio. Since most applications communicate with users via message boxes, I thought it’s time we described our WinForms Message Box controls in greater detail and show you what we have in store in our upcoming release (v20.1).
DevExpress XtraMessageBox
objects are counterparts to default WinForms messages. At first glance, the primary advantage of XtraMessageBox lies in its ability to use DevExpress application skins. Though this is an important advantage (after all, who wants to display standard gray-ish messages within a fully themed WinForms application), it is by no means the only strength of XtraMessageBox.

While you can display a message using a standard approach (pass text strings and a button set into a static XtraMessageBox.Show()
method overload), you can also create an XtraMessageBoxArgs
object and pass it as the only Show method parameter. As you might expect, this object allows you to incorporate additional behavior. For example, you can display messages that automatically close when an embedded timer expires.
XtraMessageBoxArgs args = new XtraMessageBoxArgs();
args.AutoCloseOptions.Delay = 5000;
args.Caption = "Auto-close message";
args.Text = "This message closes automatically after 5 seconds.";
args.Buttons = new DialogResult[] { DialogResult.OK, DialogResult.Cancel };
//show a countdown on a default button
args.AutoCloseOptions.ShowTimerOnDefaultButton = true;
XtraMessageBox.Show(args);
The XtraMessageBoxArgs.Showing
event allows you to access and modify a message form before it is displayed on-screen. You can use this for all sorts of things. For instance, to limit message width...

XtraMessageBoxArgs args = new XtraMessageBoxArgs();
args.Caption = "A very long message";
args.Text = "A message box attempts to show all of its text content in one line. " +
"If you do not limit the form size, this message will be very long and thin. " +
"Set the maximum form width and the form will wrap this long text.";
args.Buttons = new DialogResult[] { DialogResult.OK, DialogResult.Cancel};
args.Showing += Args_Showing;
XtraMessageBox.Show(args);
private void Args_Showing(object sender, XtraMessageShowingArgs e)
{
e.Form.MaximumSize = new Size(600, 600);
}
...or customize message buttons (change captions or supply them with vector images).
XtraMessageBoxArgs args = new XtraMessageBoxArgs();
args.Caption = "A message with icons";
args.Text = "This message displays custom SVG images in its buttons";
args.Buttons = new DialogResult[] {
DialogResult.OK, DialogResult.Cancel, DialogResult.Retry };
args.Showing += Args_Showing;
XtraMessageBox.Show(args);
void Args_Showing(object sender, XtraMessageShowingArgs e) {
foreach (var control in e.Form.Controls)
{
SimpleButton button = control as SimpleButton;
if (button != null)
{
button.ImageOptions.SvgImageSize = new Size(16, 16);
button.ImageOptions.ImageToTextAlignment = ImageAlignToText.LeftCenter;
//button.Height = 25;
switch (button.DialogResult)
{
case (DialogResult.OK):
button.ImageOptions.SvgImage = svgImageCollection1[0];
break;
case (DialogResult.Cancel):
button.ImageOptions.SvgImage = svgImageCollection1[1];
break;
case (DialogResult.Retry):
button.ImageOptions.SvgImage = svgImageCollection1[2];
break;
}
}
}
}
With v20.1, you will be able to easily include a "Do not show this message again" checkbox into your messages. If you’d like to incorporate this capability in your project, simply set the Boolean XtraMessageBoxArgs.DoNotShowAgainCheckBoxVisible
property to true. And yes, the checkbox text is also customizable.
XtraMessageBoxArgs args = new XtraMessageBoxArgs();
args.Caption = "Message";
args.Text = "You are using a trial version. The trial period expires in 30 days";
args.DoNotShowAgainCheckBoxVisible = true;
args.DoNotShowAgainCheckBoxText = "Do not remind me again";
XtraMessageBox.Show(args);
This checkbox does not do anything itself. When the message appears on-screen (or is dismissed), it raises Load
and Closed
events. You need to handle these events to store and retrieve e.Visible
property value. This property value specifies whether a user chose to suppress the message. If Load
event arguments receive false for the e.Visible property value, the message is canceled.
args.Load += Args_Load;
args.Closed += Args_Closed;
void Args_Closed(object sender, XtraMessageBoxClosedArgs e) {
//save e.Visible to a database or a local storage file
}
void Args_Load(object sender, XtraMessageBoxLoadArgs e) {
//retireve the value from a database or a local storage file
e.Visible = value;
}
Along with e.Visible, you can also store the e.DialogResult
property value. It corresponds to the last known DialogResult used when the message was closed. If a message was suppressed, you can use this value so that the Show method returns the last user choice instead of DialogResult.None.
void Args_Load(object sender, XtraMessageBoxLoadArgs e) {
e.Visible = _restoredVisibleValue_;
if (!e.Visible)
{
//restore the e.DialogResult property
e.DialogResult = _restoredDialogResultValue_;
}
}
For those who do not want to manually save and restore these parameters, we give you the option to store them in the registry. To that, call the SaveToRegistry
method on the Closed event, and RestoreFromRegistry
on Load.
void Args_Closed(object sender, XtraMessageBoxClosedArgs e) {
e.SaveToRegistry();
}
void Args_Load(object sender, XtraMessageBoxLoadArgs e) {
e.RestoreFromRegistry();
}
This code saves DialogResult and Visible keys under the Computer\HKEY_CURRENT_USER\Software\X\Y path, where:
- X - value of the
XtraMessageBox.RegistryPath
property, or Path.Combine(Application.CompanyName, Application.ProductName, "Messages") if the property is not set;
- Y - value of the
XtraMessageBoxArgs.RegistryKey
property, or an automatically generated ID.
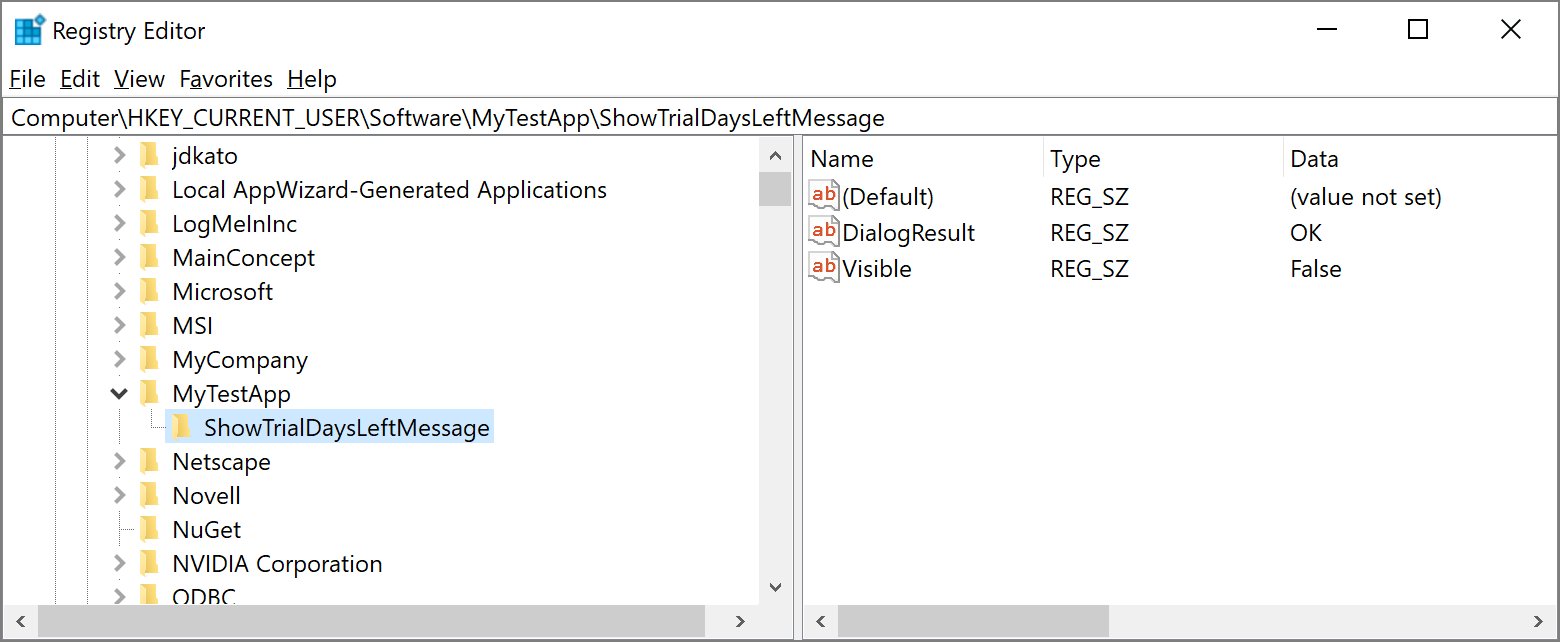
Last but not least, you can forcibly display a message even when a user has chosen to hide it. To do that, call the e.ShowMessage
method in the Load event handler. The boolean method parameter specifies whether the "Do not show again" checkbox should be checked.
XtraMessageBoxArgs args;
int trialDaysLeft;
//...
args = new XtraMessageBoxArgs();
args.Caption = "Message";
args.Text = "You are using a trial version. The trial period expires in " +
trialDaysLeft.ToString() + " days.";
args.DoNotShowAgainCheckBoxVisible = true;
args.RegistryPath = "MyTestApp";
args.RegistryKey = "ShowTrialDaysLeftMessage";
args.Load += Args_Load;
args.Closed += Args_Closed;
void Args_Closed(object sender, XtraMessageBoxClosedArgs e) {
e.SaveToRegistry();
}
void Args_Load(object sender, XtraMessageBoxLoadArgs e) {
e.RestoreFromRegistry();
if (!e.Visible && trialDaysLeft < 3) {
e.MessageBoxArgs.Text =
"Your trial expires in " + trialDaysLeft.ToString() + " day(s)!";
e.MessageBoxArgs.DoNotShowAgainCheckBoxVisible = false;
e.ShowMessage(false);
}
}
Free DevExpress Products - Get Your Copy Today
The following free DevExpress product offers remain available. Should you have any questions about the free offers below, please submit a ticket via the
DevExpress Support Center at your convenience. We'll be happy to follow-up.